iARSControls_8h-source.html
iARSControls.h
00001 /************************************************************* 00002 * 00003 * IAEM-iARS project 00004 * 00005 * File: iARSControls 00006 * 00007 * Description: Declaration for all iARSControls 00008 * 00009 * Created: 17.4.2003 00010 * Author: Christopher Frauenberger (frauenberger@iem.at) 00011 * Version: $Id: iARSControls.h,v 1.18 2004/11/25 12:55:51 frauenberger Exp $ 00012 * 00013 * Copyright (C) IEM 2003, Christopher Frauenberger [frauenberger@iem.at] 00014 * 00015 * This library is free software; you can redistribute it and/or 00016 * modify it under the terms of the GNU Lesser General Public 00017 * License as published by the Free Software Foundation; either 00018 * version 2.1 of the License, or (at your option) any later version. 00019 * 00020 * This library is distributed in the hope that it will be useful, 00021 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00022 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00023 * Lesser General Public License for more details. 00024 * 00025 * You should have received a copy of the GNU Lesser General Public 00026 * License along with this library; if not, write to the Free Software 00027 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00028 * 00029 * IEM - Institute of Electronic Music and Acoustics, Graz 00030 * Inffeldgasse 10/3, 8010 Graz, Austria 00031 * http://iem.at 00032 *************************************************************/ 00033 00034 #ifndef _IARSCONTROLS_H_ 00035 #define _IARSCONTROLS_H_ 00036 00037 #include "iARS.h" 00038 #include "iARSControlElement.h" 00039 #include <qslider.h> 00040 #include <qlabel.h> 00041 #include <qlcdnumber.h> 00042 #include <qpushbutton.h> 00043 #include <qpainter.h> 00044 #include <qdial.h> 00045 #include <qlineedit.h> 00046 #ifdef UNIX 00047 #include <qwt/qwt_plot.h> 00048 #elif WIN32 00049 #include <qwt_plot.h> 00050 #endif 00051 00052 //------------------------------------------------------------ 00053 // iARSHSlider class 00057 class iARSHSlider : public iARSControlElement { 00058 00059 Q_OBJECT 00060 00061 protected: 00063 int min; 00065 int max; 00067 QString name; 00069 QLabel *valuelabel; 00071 QSlider *slider; 00072 00073 00074 public: 00075 00077 iARSHSlider() {}; 00078 00080 iARSHSlider(QWidget* parent, const char* name) : iARSControlElement(parent,name) {} 00081 00083 iARSHSlider(QWidget*, const char*, int, int, QString); 00084 00086 void setValue(XmlRpc::XmlRpcValue); 00087 00089 void paintEvent(QPaintEvent *theEvent); 00090 00091 00092 public slots: 00093 00095 void valueChanged(int); 00096 }; 00097 00098 //------------------------------------------------------------ 00099 // iARSVSlider class 00103 class iARSVSlider : public iARSHSlider { 00104 00105 Q_OBJECT 00106 00107 public: 00108 00110 iARSVSlider(QWidget*, const char*, int, int, QString); 00111 00113 void paintEvent(QPaintEvent *theEvent); 00114 00115 00116 }; 00117 00118 //------------------------------------------------------------ 00119 // iARSButton class 00123 class iARSButton : public iARSControlElement { 00124 00125 Q_OBJECT 00126 00127 private: 00128 00130 QPushButton *button; 00131 00133 bool toggle; 00134 00135 public: 00136 00138 iARSButton() {}; 00139 00141 iARSButton(QWidget*, const char*, QString, bool); 00142 00144 void setValue(XmlRpc::XmlRpcValue); 00145 00146 00147 public slots: 00148 00150 void clicked(); 00151 }; 00152 00153 00154 //------------------------------------------------------------ 00155 // iARSLevelMeter 00159 class iARSLevelMeter : public iARSControlElement { 00160 00161 Q_OBJECT 00162 private: 00164 int min; 00166 int max; 00168 QLabel *label; 00170 class Meter : public QWidget { 00171 private: 00172 iARSLevelMeter *parent; 00173 public: 00175 Meter(iARSLevelMeter* parent) : QWidget (parent) { this->parent=parent; } 00177 void paintEvent(QPaintEvent *theEvent); 00178 }; 00180 friend class Meter; 00181 00183 Meter *meter; 00184 00185 public: 00187 iARSLevelMeter() {}; 00188 00190 iARSLevelMeter(QWidget*, const char*, int, int, QString); 00191 00193 void setValue(XmlRpc::XmlRpcValue); 00194 00195 }; 00196 00197 //------------------------------------------------------------ 00198 // iARSKnob class 00202 class iARSKnob : public iARSControlElement { 00203 00204 Q_OBJECT 00205 00206 protected: 00208 int min; 00210 int max; 00212 QLabel *label; 00214 QLabel *vlabel; 00216 QDial *dial; 00218 QLCDNumber *lcd; 00219 00220 public: 00221 00223 iARSKnob() {}; 00224 00226 iARSKnob(QWidget* parent, const char* name) : iARSControlElement(parent,name) {} 00227 00229 iARSKnob(QWidget*, const char*, int, int, QString); 00230 00232 void setValue(XmlRpc::XmlRpcValue); 00233 00234 public slots: 00235 00237 void valueChanged(int); 00238 }; 00239 00240 //------------------------------------------------------------ 00241 // iARSGLWidget class 00247 class iARSGLWidget : public iARSControlElement { 00248 00249 Q_OBJECT 00250 00251 public: 00253 iARSGLWidget(QWidget* parent, const char* name, QString binding); 00254 00256 void setValue(XmlRpc::XmlRpcValue); 00257 00258 private: 00259 00261 QString window; 00262 00264 QButton *button; 00265 00266 public slots: 00267 00269 void clicked(); 00270 }; 00271 00272 //------------------------------------------------------------ 00273 // iARSTextfield class 00279 class iARSTextfield : public iARSControlElement { 00280 00281 Q_OBJECT 00282 00283 protected: 00285 QLabel *label; 00287 QLineEdit *line; 00289 string s_value; 00290 00291 public: 00292 00294 iARSTextfield() {}; 00295 00297 iARSTextfield(QWidget* parent, const char* name) : iARSControlElement(parent,name) {} 00298 00300 iARSTextfield(QWidget*, const char*, QString); 00301 00303 void setValue(XmlRpc::XmlRpcValue); 00304 00305 public slots: 00306 00308 void returnPressed(); 00309 00310 }; 00311 00312 00313 //------------------------------------------------------------ 00314 // iARSTextdisplay class 00318 class iARSTextdisplay : public iARSTextfield { 00319 00320 Q_OBJECT 00321 00322 public: 00323 00325 iARSTextdisplay() {}; 00326 00328 iARSTextdisplay(QWidget* parent, const char* name) : 00329 iARSTextfield(parent,name) {}; 00330 00332 iARSTextdisplay(QWidget* parent, const char* bind, QString name) : 00333 iARSTextfield(parent, bind, name) {}; 00334 00336 void setValue(XmlRpc::XmlRpcValue); 00337 00338 00339 }; 00340 00341 //------------------------------------------------------------ 00342 // iARSTable class 00347 class iARSTable : public iARSControlElement { 00348 00349 Q_OBJECT 00350 00351 public: 00352 00354 iARSTable(QWidget* p, const char* n) : 00355 iARSControlElement(p,n) {}; 00356 00358 iARSTable(QWidget* p, const char* n, int size, 00359 double min, double max, QString binding); 00360 00362 void setValue(XmlRpc::XmlRpcValue); 00363 00364 private: 00365 00367 QwtPlot *plot; 00368 00370 long curve; 00371 00373 QArray<double> array; 00374 00375 typedef struct { 00376 int size; 00377 QArray<double> x; 00378 } t; 00379 00380 t xscale; 00381 00382 }; 00383 00384 #endif
Generated on Thu Nov 25 15:57:06 2004 for iARS(internetAudioRenderingSystem) by
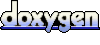